Weapon Scripting I
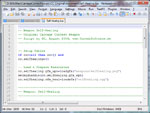
Requirements
This is a guide which explains how to script weapons for Carnage Contest with Lua. Programming/Lua knowledge is recommended for better understanding!New to Lua? You can find some tutorials here! Or just search at Google!
I recommend you to use Notepad++ as editor for Lua scripts but you are free to use any text editor you like. Just make sure that you save your scripts as plain ANSI ASCII (never use UTF-8 or other encodings!) files with ".lua" as extension.
Analyzing a Carnage Contest Weapon
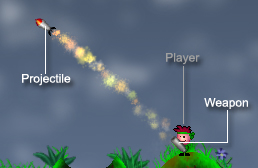
First we will have a look at a simple "weapon", which does not have any projectiles. This is the original "Self-Healing" script with some comments/effects removed for simplicity.
You can find the original script in your Carnage Contest folder @ scripts/CC Original/movement/Self-Healing.lua
-- Setup Tables if cc==nil then cc={} end cc.selfhealing={} -- Load & Prepare Ressources cc.selfhealing.gfx_wpn=loadgfx("weapons/selfhealing.png") setmidhandle(cc.selfhealing.gfx_wpn) cc.selfhealing.sfx_heal=loadsfx("selfhealing.ogg") cc.selfhealing.id=addweapon("cc.selfhealing","Self-Healing",cc.selfhealing.gfx_wpn,1,2) function cc.selfhealing.draw() -- Do nothing! end function cc.selfhealing.attack(attack) if (weapon_shots<=0) then if (attack==1) then -- No more weapon switching! useweapon(0) weapon_shots=weapon_shots+1 -- Heal playerheal(0,50) -- End Turn endturn() end end end
Setup Tables
if cc==nil then cc={} end cc.selfhealing={}
cc.selfhealing={} creates the table for the weapon in our namespace. This table will be used to store weapon functions and variables. Using the name of the weapon as name of the table is best practice.
Load & Prepare Ressources
cc.selfhealing.gfx_wpn=loadgfx("weapons/selfhealing.png") setmidhandle(cc.selfhealing.gfx_wpn) cc.selfhealing.sfx_heal=loadsfx("selfhealing.ogg")
Add Weapon
cc.selfhealing.id=addweapon("cc.selfhealing","Self-Healing",cc.selfhealing.gfx_wpn,1,2)
The command has five parameters:
- table: the Lua table which contains all variables/functions for the weapon
- name: the name for you weapon which will be displayed in-game
- icon: the image which will be used as icon for your weapon
- amount: the default amount of uses, values from 0-99 or 100 for infinite amount, or leave for infinite amount
- first use: the first round in which this weapon can be used, values from 1-10, or leave for instant use
This command is essential as said before. It's important that you use it exactly ONCE in each weapon script!
Draw Function
function cc.selfhealing.draw() -- Do nothing! end
The function has no content in this case because the self-healing 'weapon' has no images which need to be drawn!
Attack Function
function cc.selfhealing.attack(attack) if (weapon_shots<=0) then if (attack==1) then -- No more weapon switching! useweapon(0) weapon_shots=weapon_shots+1 -- Heal playerheal(0,50) -- End Turn endturn() end end end
First the function checks if the global variable weapon_shots is less or equal zero. In other words it checks if the 'weapon' has been fired already.
Global Weapon Variables
weapon_shots is one of 7 global weapon variables which will be reset to 0 automatically by the game when you select another weapon or when the turn ends. Here's a short list:- weapon_shots: commonly used for the amount of fired shots
- weapon_charge: used for the charge power value (for weapons like bazooka or grenade)
- weapon_mode: use it when your weapon has different modes or something like that
- weapon_timer: for timing stuff e.g. for the delay between two shots
- weapon_position: used by hudpositioning command. Is 1 if player specified a position on the map - else 0
- weapon_x: used by hudpositioning. X coordinate the player specified
- weapon_y: used by hudpositioning. Y coordinate the player specified
Attack / Use Weapon / End Turn
After checking if the weapon has been fired/used already, the script checks if space is pressed (if attack==1). So if the weapon has NOT been used yet AND if space is pressed, something will happen.The command useweapon is called. It decreases the amount in the weapon inventory of the current team by 1 (if the amount is limited). The parameter 0 tells the game to close the weapon selection menu. You will not be able to use other weapons after using this one. Use 1 as parameter if you want to allow to use more weapons this turn.
Next weapon_shots is increased by 1. Together with the condition at the beginning of this function this will make sure that the weapon will only be used once.
Afterwards playerheal(0,50) is called. It gives 50 healtpoints to the player with ID 0. ID 0 is not a real ID but a shortcut. It is equal to the ID of the player who is currently controlled. Instead you could also use playercurrent() to get the ID of the current player. So remember: 0 as player ID in player functions always means that the command is used on the current player!
Finally the last command is endturn. This commands ends the turn. Ending a turn means that the backing time will start. The current player will not be able to attack anymore and soon the control will be given to the next player.
Continue to Part II